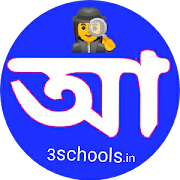
Apu
June 07, 2024 ›
#HowTo
›
#Javascript
❌
How To Get Selected Radio Button Value In Javascript
In this article, you are going to learn how to get the selected radio button value in JavaScript. Radio buttons are a common form element that are used to allow users to select one option from a group of options. We will explore three approach to achieve this using JavaScript.
Approach 1: Using the 'checked' Property and a Loop
This method involves iterating through all the radio buttons and checking which one is selected using the checked property.
<input type="radio" name="color" value="red" id="red"> Red <input type="radio" name="color" value="green" id="green"> Green <input type="radio" name="color" value="blue" id="blue"> Blue <button onclick="getSelectedValue()">Get Selected Value</button> <p id="result"></p> <script> function getSelectedValue() { var radios = document.getElementsByName('color'); for (var i = 0; i < radios.length; i++) { if(radios[i].checked) { document.getElementById('result').innerHTML = radios[i].value; break; } } } </script>
Explanation
- The getElementsByName method is used to get all the radio buttons with the name "color".
- A loop is used to iterate through the radio buttons and check which one is selected using the checked property.
- The selected value is displayed in a paragraph element with the id "result".
- The break statement is used to exit the loop once the selected value is found.
Approach 2: Using the 'querySelector' Method
This method involves using the querySelector method to select the checked radio button.
<input type="radio" name="color" value="red" id="red"> Red <input type="radio" name="color" value="green" id="green"> Green <input type="radio" name="color" value="blue" id="blue" checked=""> Blue <button onclick="getSelectedValue()">Get Selected Value</button> <p id="result"></p> <script> function getSelectedValue() { var selectedValue = document.querySelector('input[name="color"][checked]').value; document.getElementById('result').innerHTML = selectedValue; } </script>
- The querySelector method is used to select the checked radio button with the name "color".
- The value property is used to get the selected value and displayed in a paragraph element with the id "result".
It is supported in modern browsers, but may not work in older browsers.
Approach 3: Using an Event Listener
This method involves adding an event listener to each radio button to detect when it is selected.
<input type="radio" name="color" value="red" id="red"> red <input type="radio" name="color" value="green" id="green"> green <input type="radio" name="color" value="blue" id="blue"> Blue <p id="result"></p> <script> var radios = document.getElementsByName('color'); for (var i = 0; i < radios.length; i++) { radios[i].addEventListener('change', function() { document.getElementById('result').innerHTML = this.value; }); } </script>
Explain
- An event listener is added to each radio button to detect when it is selected.
- The change event is used to trigger the event listener.
- The this keyword is used to refer to the selected radio button.
- The selected value is displayed in a paragraph element with the id "result".
This method is more dynamic and efficient than the previous methods.
save
listen
AI Answer
How To Get Selected Radio Button Value In Javascript
0
In this article, you are going to learn how to get the selected radio button value in Jav…
asked
Apu
0 answers
2915
In this article, you are going to learn how to get the selected radio button value in Jav…
Answer Link
answered
Apu