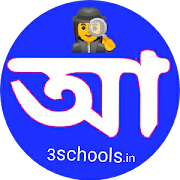
How to check or uncheck radio button dynamically using JavaScript?
In this article, we will explore how to dynamically check or uncheck radio buttons using JavaScript. We will cover two different methods for achieving this, and provide complete code snippets for each approach.
Approach 1: Using the checked property
The checked property is a boolean attribute that can be used to set or retrieve the checked state of a radio button. To check or uncheck a radio button dynamically using this property, we can simply set its value to true or false, respectively.
Here is an example of how to use the checked property to check a radio button.
<input type="radio" id="option1" name="options"> <label for="option1">Option 1</label> <script> const radioButton = document.getElementById("option1"); radioButton.checked = true; </script>
Explanation:
- We create a radio button with the id attribute set to "option1" and the name attribute set to "options".
- We retrieve the radio button using the document.getElementById() method and store it in the radioButton variable.
- Then we set the checked property of the radio button to true using the assignment operator (=).
Approach 2: Using the setAttribute() method
The setAttribute() method is a built-in method that allows us to set the value of an attribute for a given element. To check or uncheck a radio button dynamically using this method, we can set the value of the checked attribute to true or false, respectively.
Here is an example of how to use the setAttribute() method to check a radio button.
<input type="radio" id="option2" name="options"> <label for="option2">Option 2</label> <script> const radioButton = document.getElementById("option2"); radioButton.setAttribute("checked", "true"); </script>
Explanation:
- We create a radio button with the id attribute set to "option2" and the name attribute set to "options".
- We retrieve the radio button using the document.getElementById() method and store it in the radioButton variable.
- Then we set the checked attribute of the radio button to true using the setAttribute() method.
How to uncheck radio button using JavaScript?
To uncheck a radio button dynamically, we can simply set the checked property or attribute to false. Here is an example of how to uncheck a radio button using the checked property.
<input checked="" type="radio" id="option1" name="options"> <label for="option1">Option 1</label> <button id="uncheckButton">Uncheck Radio Button</button> <script> const radioButton = document.getElementById("option1"); const uncheckButton = document.getElementById("uncheckButton"); uncheckButton.addEventListener("click", function() { radioButton.checked = false; }); </script>
Conclusion
In this article, we have explored two different methods for dynamically checking or unchecking radio buttons using JavaScript. These methods include using the checked property and the setAttribute() method.