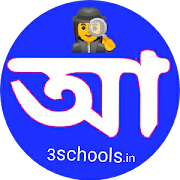
Apu
May 03, 2024 .
#HowTo
.
#Javascript
❌
How can I detect if the internet connection is offline in JavaScript?
In this article, we'll explore two approaches to detect if the internet connection is offline or online in JavaScript.
Approach 1: Using the navigator.onLine Property
JavaScript provides a built-in property navigator.onLine to check the online status of the browser. This property returns a boolean value indicating whether the browser is in online mode or not.
<script> const isOnline = navigator.onLine; if(!isOnline) { console.log("You are currently offline."); } else { console.log("You are online."); } </script>
Explanation :
- Access the navigator.onLine property to check the online status.
- Assign the boolean value returned by navigator.onLine to a variable.
- Use a conditional statement to determine if the browser is online or offline.
- Display appropriate messages based on the online status.
Approach 2: Using the "offline" and "online" Events
JavaScript also provides offline and online events that fire when the browser transitions between online and offline states. You can listen for these events to perform actions accordingly.
<script> window.addEventListener("offline", () => { console.log("You are now offline."); }); window.addEventListener("online", () => { console.log("You are now online."); }); </script>
- Attach event listeners to the window object for the offline and online events.
- Define callback functions to execute when these events are triggered.
- Log appropriate messages indicating the transition between online and offline states.
save
listen
AI Answer
How can I detect if the internet connection is offline in JavaScript?
0
In this article, we'll explore two approaches to detect if the internet connection is…
asked
Apu
0 answers
2915
In this article, we'll explore two approaches to detect if the internet connection is…
Answer Link
answered
Apu