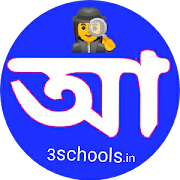
Apu
May 06, 2024 ›
#HowTo
›
#Javascript
❌
How can I auto filter a HTML selectlist using JavaScript?
In this blog post, we'll explore how to implement auto-filtering functionality for HTML select lists using JavaScript.
First, let's create a simple HTML select list with some options:
<select id="mySelect"> <option value="1">Apple</option> <option value="2">Banana</option> <option value="3">Cherry</option> <option value="4">Orange</option> <option value="5">Pineapple</option> </select>
Next, we will add an <input> field where users can type to filter the select list:
<input type="text" id="myInput" onkeyup="filterList()" placeholder="Search for fruits...">
Now, let's write the JavaScript function filterList() that will filter the select list based on the user input:
<script> function filterList() { let input, filter, select, options, option, i, txtValue; input = document.getElementById("myInput"); filter = input.value.toUpperCase(); select = document.getElementById("mySelect"); options = select.getElementsByTagName("option"); for (i = 0; i < options.length; i++) { option = options[i]; txtValue = option.textContent || option.innerText; if(txtValue.toUpperCase().indexOf(filter) > -1) { option.style.display = ""; } else { option.style.display = "none"; } } } </script>
- The filterList() function is defined to handle the filtering of options in the select list based on user input.
- The function first retrieves the user input from the input field and converts it to uppercase for case-insensitive filtering.
- Then it accesses the select list and gets all the option elements within it.
- For each option, the function compares the text content of the option with the user input. If the text content contains the filter text, only then the option is displayed; otherwise, it is hidden.
- This allows the select list to dynamically update and show only the options that match the user's input as they type in the input field.
Example :
Now, when a user types in the input field, the select list will automatically filter out options that do not match the input.
save
listen
AI Answer
How can I auto filter a HTML selectlist using JavaScript?
1
In this blog post, we'll explore how to implement auto-filtering functionality for HT…
asked
Apu
1 answers
2915
In this blog post, we'll explore how to implement auto-filtering functionality for HT…
Answer Link
answered
Apu
<script>
const selectList = document.getElementById('selectList');
const filterInput = document.getElementById('filterInput');
filterInput.addEventListener('input', function() {
const filterValue = this.value.toLowerCase();
Array.from(selectList.options).forEach(option =>{
option.style.display = option.textContent.toLowerCase().includes(filterValue) ? 'block' : 'none';
});
});
</script>
Try It Yourself