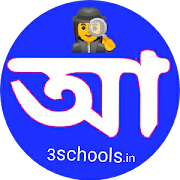
Apu
April 15, 2024 .
#HowTo
.
#Javascript
❌
Remove all the child elements of a DOM node in JavaScript
I'm working on a web project where I need to remove all the child elements of a specific DOM node using JavaScript. Can anyone guide me on how to accomplish this task?
<div id="container"> <p>This is the first paragraph.</p> <p>This is the second paragraph.</p> <p>This is the third paragraph.</p> </div> <button onclick="removeChildElements()">Remove All Child Elements</button>
save
listen
AI Answer
Remove all the child elements of a DOM node in JavaScript
3
I'm working on a web project where I need to remove all the child elements of a speci…
asked
Apu
3 answers
2915
I'm working on a web project where I need to remove all the child elements of a speci…
Answer Link
answered
Apu
One simple approach is to set the innerHTML property of the container element to an empty string. This effectively removes all the child elements inside the container.
<script>
functionremoveChildElements() {
var container = document.getElementById('container');
container.innerHTML = '';
}
</script>
This code snippet clears out all the HTML content within the container div when the button is clicked.
You can loop through each child node of the container and remove them one by one.
<script>
functionremoveChildElements() {
var container =
document.getElementById('container');
while (container.firstChild) {
container.removeChild(container.firstChild);
}
}
</script>
This code iterates over each child node of the container div and removes them until no child nodes remain. It's a more explicit way of removing child elements.
Another approach involves using the removeChild() method in a loop to remove each child node.
<script>
functionremoveChildElements() {
var container =
document.getElementById('container');
var child = container.lastElementChild;
while (child) {
container.removeChild(child);
child = container.lastElementChild;
}
}
</script>