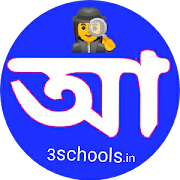
Apu
April 12, 2024 ›
#HowTo
›
#Javascript
❌
How can I remove a child node in HTML using JavaScript?
I'm trying to manipulate the DOM in my web project, and I need to remove a specific child node from an HTML element using JavaScript.
Can someone guide me on how to do this? Here's the code snippet I'm working with:
<div id="parent"> <p>First child</p> <p>Second child</p> <p>Third child</p> </div>
And here's what I've tried so far in my JavaScript.
<script> const parent = document.getElementById("parent"); const childToRemove = parent.querySelector("p:nth-child(2)"); parent.removeChild(childToRemove); </script>
However, it's not working as expected. Can anyone provide a solution with an explanation of the code?
save
listen
AI Answer
How can I remove a child node in HTML using JavaScript?
6
I'm trying to manipulate the DOM in my web project, and I need to remove a specific c…
asked
Apu
6 answers
2915
I'm trying to manipulate the DOM in my web project, and I need to remove a specific c…
Answer Link
answered
Apu
const parent = document.getElementById("parent");
const childToRemove = parent.querySelector("p:nth-child(2)");
if (childToRemove) {
parent.removeChild(childToRemove);
}
</script>
First, we retrieve the parent element using getElementById. Then, we use querySelector to select the second <p> element within the parent. We check if the childToRemove exists to avoid errors, then remove it using removeChild if it's found.
const parent = document.getElementById("parent");
const childToRemove = parent.children[1];
parent.removeChild(childToRemove);
</script>
We access the children of the parent element using the children property, which returns a collection of all child elements. Since arrays are zero-indexed, children[1] refers to the second child element. We then remove this child from the parent using removeChild.
const parent = document.getElementById("parent");
const childToRemove = parent.children[1];
if (childToRemove) {
parent.removeChild(childToRemove);
}
</script>
Here, we first ensure that childToRemove exists before attempting to remove it from the parent element. This prevents errors in case the specified child doesn't exist.
<script>
const parent = document.getElementById("parent");
const childToRemove = parent.children[1];
if (childToRemove) {
childToRemove.remove();
}
</script>
const parent = document.getElementById("parent");
parent.removeChild(parent.children[1]);
<script>
const parent = document.getElementById("parent");
Array.from(parent.children).forEach(child =>{
if (child.textContent === "Second child") {
parent.removeChild(child);
}
});
</script>