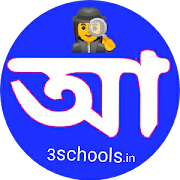
Apu
March 23, 2024 ›
#Javascript
›
#loop
❌
What is the best way to break from nested loops in JavaScript?
Breaking from nested loops in JavaScript can be tricky, but there are several effective methods to accomplish this task. One commonly used approach is by utilizing labels with the break statement. Here's the example:
<script> // Define a label for the outer loop outerLoop: for (let i = 0; i < 5; i++) { for (let j = 0; j < 5; j++) { if (i === 2 && j === 2) { // Break out of both loops when condition is met break outerLoop; } console.log(`i: ${i}, j: ${j}`); } } </script>
In this example, outerLoop is a label assigned to the outer loop. When the break statement is encountered with the outerLoop label, it breaks out of both the inner and outer loops.
save
listen
AI Answer
What is the best way to break from nested loops in JavaScript?
2
Breaking from nested loops in JavaScript can be tricky, but there are several effective m…
asked
Apu
2 answers
2915
Breaking from nested loops in JavaScript can be tricky, but there are several effective m…
Answer Link
answered
Apu
Instead of labels, you can use a variable to control the loop execution. Set the variable's value to true when you want to break from the loops.
<script>
let shouldBreak = false;
for (let i = 0; i < 5; i++) {
for (let j = 0; j < 5; j++) {
if (i === 2 && j === 2) {
shouldBreak = true;
break;
}
console.log(`i: ${i}, j: ${j}`);
}
if (shouldBreak) {
break;
}
}
</script>
Wrap your nested loops inside a function and use the return statement to exit the function when needed.
<script>
function breakFromLoops() {
for (let i = 0; i < 5; i++) {
for (let j = 0; j < 5; j++) {
if (i === 2 && j === 2) {
return;
}
console.log(`i: ${i}, j: ${j}`);
}
}
}
breakFromLoops();
</script>