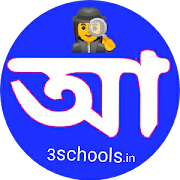
Apu
February 03, 2024 .
#HowTo
.
#Javascript
❌
How to convert decimal to hexadecimal in JavaScript
In this article, we'll explore two methods to convert decimal to hexadecimal in JavaScript. We'll discuss and provide code examples for each method.
Convert decimal to hexadecimal using toString() method
Converting decimal to hexadecimal using the toString() method is straightforward in JavaScript. Here's how you can do it:
<script> // Decimal number const decimalNumber = 255; // Convert decimal to hexadecimal const hexadecimal = decimalNumber.toString(16); // Output the hexadecimal value console.log("Hexadecimal:", hexadecimal); </script>
- Define a decimal number to convert.
- Use the toString() method with a base of 16 to convert the decimal number to hexadecimal.
- Output the hexadecimal value using console.log().
Using the Number.prototype.toString() Method
Another method to convert decimal to hexadecimal is by using the Number.prototype.toString() method. Here's how to do it:
<script> // Decimal number const decimalNumber = 255; // Convert decimal to hexadecimal const hexadecimal = (decimalNumber).toString(16); // Output the hexadecimal value console.log("Hexadecimal:", hexadecimal); </script>
- Define a decimal number to convert.
- Use the Number.prototype.toString() method with a base of 16 to convert the decimal number to hexadecimal.
- Output the hexadecimal value using console.log().
Conclusion
In this article, we explored two methods to convert decimal to hexadecimal in JavaScript. The first method utilizes the toString() method directly on the decimal number, while the second method uses the Number.prototype.toString() method.
save
listen
AI Answer
How to convert decimal to hexadecimal in JavaScript
2
In this article, we'll explore two methods to convert decimal to hexadecimal in JavaS…
asked
Apu
2 answers
2915
In this article, we'll explore two methods to convert decimal to hexadecimal in JavaS…
Answer Link
answered
Apu
<script>
const decimalNumber = 255;
const hexadecimal = decimalNumber.toString(16);
console.log("Hexadecimal:", hexadecimal);
</script>
Use the toString() method with a base of 16 to convert the decimal number to hexadecimal.
<script>
const decimalNumber = 255;
const hexadecimalNumber = decimalNumber.toString(16);
console.log(hexadecimalNumber);
</script>
The 16 passed as an argument specifies that the conversion should be to base 16 (hexadecimal).