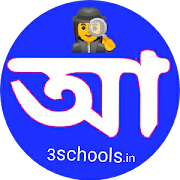
Apu
January 19, 2024 .
#HowTo
.
#Javascript
❌
How to Convert a String to a Number in JavaScript
In this article, we are going to explore various methods to convert a string into a number using JavaScript. Let's dive into four different approaches to achieve this task.
Convert String Into Number Using Parseint() Method
The parseInt() function is a fundamental method to convert a string to an integer in JavaScript.
<script> const str = "123"; const num = parseInt(str); console.log(num) </script>
1. Use parseInt() function.
2. Pass the string as an argument.
3. Assign the result to a variable.
Convert String To Number Using parsefloat() Method
If your string contains decimal numbers, parseFloat() is the go-to method.
<script> const str = "123.45"; const num = parseFloat(str); console.log(num) </script>
- Utilize parseFloat() function.
- Provide the string with decimal values.
- Store the result in a variable and console it.
How To Convert String To Number Using The Number() Method
The Number() method is a versatile way to convert strings to both integers and floats.
<script> const str = "123.45"; const num = Number(str); console.log(num) </script>
- Use the Number() method.
- Pass the string to be converted.
- Store the result in a variable.
Using the parseFloat() with Math.floor() method
Combining parseFloat() with Math.floor() ensures you get the integer part of a string with a floating-point number.
<script> const str = "123.45"; const num = Math.floor(parseFloat(str)); console.log(num) </script>
- Use parseFloat() method to handle decimals.
- Apply Math.floor() to round down.
- Store the result in a variable.
Conclusion:
In this article, you learned four different methods to convert a string into a number in JavaScript.
save
listen
AI Answer
How to Convert a String to a Number in JavaScript
2
In this article, we are going to explore various methods to convert a string into a numbe…
asked
Apu
2 answers
2915
In this article, we are going to explore various methods to convert a string into a numbe…
Answer Link
answered
Apu
<!DOCTYPE html>
<html>
<body>
<script>
var str = "42";
var num = Number.parseInt(str);
</script>
</body>
</html>
Step 1 : Utilize Number.parseInt() method.
Step 2 : Pass the string to be converted.
Step 3 : Store the result in a variable.
Combining the unary plus operator with the Number() constructor is another concise way to convert strings to numbers.
<script>
var str = "123";
var num = Number(+str);
</script>