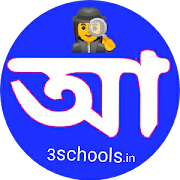
Apu
January 19, 2024 ›
#HowTo
›
#Javascript
❌
Window onload not working on refresh
I'm having a problem with the window.onload event in JavaScript. I'm trying to execute some code when the page loads, but it doesn't seem to work when I refresh the page.
<script>
window.onload = function() {
// My code here
alert("Page loaded!");
};
</script>
This works fine when I initially load the page, but when I refresh it, the code doesn't run. Can anyone help me figure out why this is happening?
save
listen
AI Answer
Window onload not working on refresh
7
I'm having a problem with the window.onload event in JavaScript. I'm trying to ex…
asked
Apu
7 answers
2915
I'm having a problem with the window.onload event in JavaScript. I'm trying to ex…
Answer Link
answered
Apu
To make your code run on page refresh, you can use the DOMContentLoaded event instead. Here's how you can modify your code:
document.addEventListener("DOMContentLoaded", function() {
// Your code here
alert("Page loaded or refreshed!");
});
document.addEventListener("DOMContentLoaded", () =>{
// Your code here
alert("Page loaded or refreshed!");
});
document.addEventListener("DOMContentLoaded", () =>{
// Your one-time code here
alert("This will run only once on load or refresh!");
}, { once: true });
This way, you won't need to manually remove the event listener afterward.
function runOnce() {
// Your first one-time code here
alert("This will run only once on load or refresh!");
// Your second one-time code here
console.log("This too will run only once on load or refresh!");
}
document.addEventListener("DOMContentLoaded", runOnce, { once: true });
This way, you can add as many functions as you need to the runOnce function, and they will all execute only once on page load or refresh.