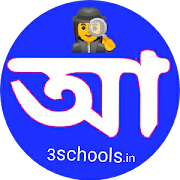
Apu
January 19, 2024 .
#HowTo
.
#Javascript
❌
How to load a text file in javascript from another file
In this article, you are going to learn two methods for loading a text file in JavaScript from another file.
Method 1: Using the FileReader API
The FileReader API in JavaScript provides a convenient way to read the contents of a file.
- Create an HTML input element: Start by creating an HTML input element to allow the user to select a file.
- Listen for the change event: Add an event listener to the input element to trigger when the user selects a file.
- Read the file: In the event handler, use the FileReader API to read the selected file.
- Access the file content: Once the file is read, you can access its content using the result property of the FileReader object.
<input type="file" id="fileInput"> <script> document.getElementById('fileInput').addEventListener('change', function () { const file = this.files[0]; const reader = new FileReader(); reader.onload = function (e) { const fileContent = e.target.result; console.log(fileContent) }; reader.readAsText(file); }); </script>
Method 2: Fetching Text Files Using the Fetch API
Another method to load text files is by using the Fetch API, which is commonly used for making network requests. You can use it to load text files from another file in a similar way.
- Use the fetch() function: Call the fetch() function with the path to the text file as an argument.
- Handle the response: Use the .then() method to handle the response and read the file as text.
- Access the file content: Once the file is fetched, you can access its content in the .then() block.
<script> fetch('/robots.txt') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.text(); }) .then(data => { console.log(data) }) .catch(error => { console.error('There was a problem with the fetch operation: ' + error.message); }); </script>
Conclusion:
In this article, you've learned two methods for loading a text file in JavaScript from another file. You can use the FileReader API for local file selection or the Fetch API for fetching text files from a remote source.
save
listen
AI Answer
How to load a text file in javascript from another file
0
In this article, you are going to learn two methods for loading a text file in JavaScript…
asked
Apu
0 answers
2915
In this article, you are going to learn two methods for loading a text file in JavaScript…
Answer Link
answered
Apu