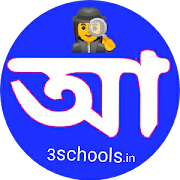
Apu
January 19, 2024 ›
#HowTo
›
#Javascript
❌
How to Convert String to Title Case with JavaScript
In this article, you are going to learn how to convert a string into Title Case using JavaScript.
What is Title Case?
Title Case is commonly used in text formatting, making the first letter of each word in a sentence uppercase, while all other letters are lowercase.
This can be handy for presenting titles, names, or other textual content in a more visually appealing manner.
Using JavaScript Split and Join method
<script> function toTitleCase(str) { return str.split(' ').map(word => word.charAt(0).toUpperCase() + word.slice(1).toLowerCase()).join(' '); } const inputString = "convert string to title case with javascript"; const titleCaseString = toTitleCase(inputString); console.log(titleCaseString); </script>
Example Explanation :
- We define a function toTitleCase that takes a string as input.
- Inside the function, we split the string into an array of words using the split(' ') method.
- Then, we use map to iterate through each word in the array and capitalize the first letter using word.charAt(0).toUpperCase().
- We convert the rest of the word to lowercase using word.slice(1).toLowerCase().
- Finally, we join the modified words back into a single string using the join(' ') method.
Convert String to Title Case Using Regular Expressions
<script> function toTitleCaseRegex(str) { return str.replace(/\w\S*/g, function(txt){return txt.charAt(0).toUpperCase() + txt.substr(1).toLowerCase();}); } const inputString = "convert string to title case with javascript"; const titleCaseString = toTitleCaseRegex(inputString); console.log(titleCaseString); </script>
Explanation:
- We define a function toTitleCaseRegex that takes a string as input.
- Inside the function, we use the replace() method with a regular expression to match words.
- The regular expression \w\S matches words in the string.
- The replace method's callback function is used to capitalize the first letter of each word and convert the rest to lowercase.
Conclusion:
In this article, you have learned two methods to convert a string to Title Case using JavaScript. Method 1 uses the split and join functions, while Method 2 leverages regular expressions with the replace function.
save
listen
AI Answer
How to Convert String to Title Case with JavaScript
0
In this article, you are going to learn how to convert a string into Title Case using Jav…
asked
Apu
0 answers
2915
In this article, you are going to learn how to convert a string into Title Case using Jav…
Answer Link
answered
Apu