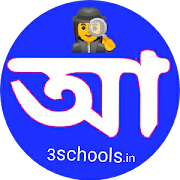
Apu
January 19, 2024 ›
#HowTo
›
#Javascript
❌
How do you performance test javascript code example?
Hey folks, I've been trying to figure out how to performance test my JavaScript code, and I could use some help.
I've got a specific piece of code that I want to optimize. Here's the code snippet I'm working with:
<script>
function calculateSum(n) {
let sum = 0;
for (let i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
</script>
This function calculates the sum of integers from 1 to n. Now, I want to find out how efficient it is, especially for large values of n.
Any suggestions on how I can measure its performance, and what tools or techniques I should use for this?
save
listen
AI Answer
How do you performance test javascript code example?
6
Hey folks, I've been trying to figure out how to performance test my JavaScript code,…
asked
Apu
6 answers
2915
Hey folks, I've been trying to figure out how to performance test my JavaScript code,…
Answer Link
answered
Apu
function calculateSum(n) {
const start = performance.now();
let sum = 0;
for (let i = 1; i <= n; i++) {
sum += i;
}
const end = performance.now();
console.log(`Execution time: ${end - start} milliseconds`);
return sum;
}
This code snippet will log the execution time in milliseconds to the console. You can then run your function with different values of n to see how it performs.
https://www.3schools.in/p/embed.html?q=CjxzY3JpcHQ+CiBmdW5jdGlvbiBjYWxjdWxhdGVTdW0obikgewogIGNvbnNvbGUudGltZSgnY2FsY3VsYXRlU3VtJyk7CiAgbGV0IHN1bSA9IDA7CiAgZm9yIChsZXQgaSA9IDE7IGkgPD0gbjsgaSsrKSB7CiAgICBzdW0gKz0gaTsKICB9CiAgY29uc29sZS50aW1lRW5kKCdjYWxjdWxhdGVTdW0nKTsKICByZXR1cm4gc3VtOwp9Cjwvc2NyaXB0Pg==
This will give you even more information about how long the execution takes for different values of n.