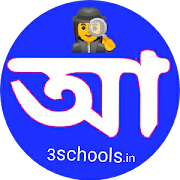
How to Stop, Break or Exit forEach loop in JavaScript
Since forEach() is a function rather than a loop, There is no way to stop or break a forEach() loop, using the break statement is a syntax error.
let numberArr = [1,2,3,4,5]; numberArr.forEach(number =>{ if(number == 4){ break; } })
If we try the above example, we will get the Illegal break statement forEach error.
Don't worry, in this article we'll look at three alternative ways to break a loop when a condition is met.
[1] Using a Local Variable. #
To break a forEach() loop in a satisfied condition, we can declare a local variable skipId at the beginning of the forEach() callback. If we set skipId to true, the forEach() callback returns immediately.
Example : stop forEach() loop in Js #
<script>
let skipId = false;
let numberArr = [1,2,3,4,5];
numberArr.forEach(number =>{
if(skipId){
return;
}
if(number > 3){
skipId = true;
return;
}
console.log(number)
})
</script>
[2] Using a for…of Loop #
We may use the for…of loop instead of forEach() method. Use the break keyword to exit the for…of loop.
Example : exit for…of loop using the break keyword. #
<script>
let numberArr = [1,2,3,4,5];
for(number of numberArr){
if(number == 3){
break;
}
console.log(number)
}
</script>
[3] Using the every() method. #
The every() method returns true if the function returns true for all elements. If the function returns false for one element, the every() method returns false.
Example : stop a loop using the every() method. #
<script>
let numberArr = [1,2,3,4,5];
numberArr.every(number =>{
if(number == 3){
return false;
}
console.log(number)
return true;
})
</script>