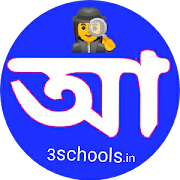
JavaScript console.log All Details With Example
The console is an object and the log() is a method.
Demo : console.log() hello world. #
<script>
console.log("Hello World")
</script>
// output => Hello World
The log() method is available in almost browsers and also JavaScript editor. The log() method is used to display anything passed into it as a parameter to the console panel. console.log() is generally used for testing purposes during development to find any errors in your code.
To access the log() method, the console object is used with a dot-operator.
Syntax #
console.log(message)
List of the all methods or functions inside the console object.

Pass a variable to the console.log() method. #
If you pass a variable to the console.log() method as a parameter, the output will be it.
Demo : Passing a variable to the log() method. #
<script>
const a = "Hello World";
console.log(a);
</script>
// output => Hello World
Pass a string to the log() method. #
If you pass a string to the log() method as a parameter, it will return the following output.
Demo : passing a string as a parameter. #
<script>
console.log("My Schools");
</script>
// output => My Schools
Pass a number to the log() method. #
In this example, I have passed a number to the log() method as a parameter.
Demo : passing a number as a parameter. #
<script>
console.log(3);
</script>
// output => 3
Demo : passing a function to the console.log() method. #
function a(){ return 5 + 5}
console.log(a());
// output => 10
Pass an object to the console.log() method. #
<script>
const color = {
name : "red",
code: "#ff0000"
}
console.log(color)
</script>
🔽Object
name:"red"
code:"#ff0000"
Example : passing an array as a parameter. #
<script>
const color = [
"red",
"#ff0000"
]
console.log(color)
</script>
🔽Array
0:"red"
1:"#ff0000"
length:2
Browser Support #
console.log() is supported in all browsers.
Chrome | IE | Edge | Firefox | Safari | Opera |
Yes | 8-11 | Yes | Yes | Yes | Yes |