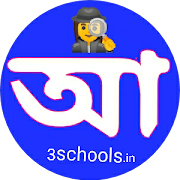
Apu
March 16, 2022 .
#HowTo
.
#Javascript
❌
How to change the content of a textarea using JavaScript
There are many methods to change the content of a textarea using javascript. In this article, I am sharing some of these methods.
Example
Try it Yourself »
Method 1 :- using innerHTML property.
For the first method, we will create a textarea with an Id attribute named my-textarea. Then create a button with onclick event named changText() because whenever a user clicks the button a function will be called. Finally, create the function and inside that function select the textarea with the id my-textarea and set its value using innerHTML property.
Example using innerHTML property.
<textarea id="my-textarea">This is a textarea.</textarea> <button onclick="changeText()">Change The Content</button> <script> function changeText(){ document.getElementById("my-textarea").innerHTML="The content is changed"; } </script>Try it Yourself »
Method 2 :- using value property.
In this method, I'm going to use value property instead of innerHTML property.
Example using value property.
<textarea id="my-textarea">This is a textarea.</textarea> <button onclick="changeText()">Change The Content</button> <script> function changeText(){ document.getElementById("my-textarea").value="The content is changed"; } </script>Try it Yourself »
Method 3 :- using innerText property.
In this method, use innerText property to change the text of a textarea. The example is given below.
Example using innerText property.
<textarea id="my-textarea">This is a textarea.</textarea> <button onclick="changeText()">Change The Content</button> <script> function changeText(){ document.getElementById("my-textarea").innerText="The content is changed"; } </script>Try it Yourself »
Method 4 :- Create a textarea dynamically using javascript
Let's have some fun. Create a textarea dynamically and add some text to it.
Create a textarea dynamically.
// Create element const textarea = document.createElement("textarea"); textarea.innerText = "This is a textarea."; // Append to body document.body.appendChild(textarea);Try it Yourself »
save
listen
AI Answer
How to change the content of a textarea using JavaScript
0
There are many methods to change the content of a textarea using javascript. In this arti…
asked
Apu
0 answers
2915
There are many methods to change the content of a textarea using javascript. In this arti…
Answer Link
answered
Apu