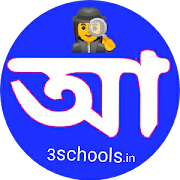
Apu
May 02, 2024 ›
#HowTo
›
#Javascript
❌
How to combine two regex in JavaScript?
In this article, we'll delve into combining two regular expressions in JavaScript. We'll explore a straightforward approach using string concatenation with HTML and JavaScript code.
Combining Regex with String Concatenation
To combine two regular expressions in JavaScript, we can use string concatenation to merge their patterns into a single regex pattern string.
Let's consider an example where we want to match email addresses that end with either .com or .org.
<script> const regex1 = /\w+@\w+\.(?:com)/; const regex2 = /\w+@\w+\.(?:org)/; const combinedRegexPattern = `${regex1.source}|${regex2.source}`; const combinedRegex = new RegExp(combinedRegexPattern, 'i'); const email = '3schools.in@gmail.com'; console.log(combinedRegex.test(email)); // Output: true </script>
Explanation
- Declare two regular expressions regex1 and regex2 to match email addresses ending with .com and .org.
- Combine their patterns using string concatenation with the | operator.
- Create a new regex object combinedRegex using the combined pattern and specify the i flag for case-insensitive matching.
- Test the combined regex pattern against an email address to verify it's working or not.
Example :
<h1>Email Validation</h1> <input type="text" id="emailInput" placeholder="Enter Your Email"> <button onclick="validateEmail()">Validate</button> <p id="result"></p> <script> function validateEmail() { const emailInput = document.getElementById('emailInput').value; const regex1 = /\w+@\w+\.(?:com)/; const regex2 = /\w+@\w+\.(?:org)/; const combinedRegexPattern = `${regex1.source}|${regex2.source}`; const combinedRegex = new RegExp(combinedRegexPattern, 'i'); const result = combinedRegex.test(emailInput) ? 'Valid email' : 'Invalid email'; document.getElementById('result').innerText = result; } </script>
Explained
- We provide an input field for users to enter an email address.
- By clicking the Validate button, the validateEmail() function is called.
- Inside this function, we retrieve the email input value and validate it against the combined regex pattern.
- The result is displayed below the input field, indicating whether the email is valid or not.
save
listen
AI Answer
How to combine two regex in JavaScript?
0
In this article, we'll delve into combining two regular expressions in JavaScript. We…
asked
Apu
0 answers
2915
In this article, we'll delve into combining two regular expressions in JavaScript. We…
Answer Link
answered
Apu