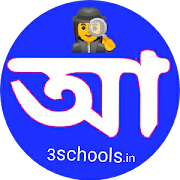
How to display a message when a button is clicked in JavaScript?
In this article, you will learn how to create a simple functionality in JavaScript that displays a message when a button is clicked. We'll explore two different methods to achieve this.
Method 1: Using HTML Attribute #
You can achieve this by adding an onclick attribute directly to the HTML button element. This attribute specifies the JavaScript code that should be executed when the button is clicked.
Explanation:
- We create a button element with an id attribute of myButton.
- The onclick attribute is set to call the displayMessage() function when the button is clicked.
- Inside the <script> tag, we define the displayMessage() function which displays an alert with the message Button was clicked!.
Method 2: Using JavaScript Event Listeners #
Alternatively, you can use JavaScript to add an event listener to the button. This approach separates the HTML and JavaScript code more cleanly.
Explanation:
- We create a button element with the same myButton id.
- In the <script> tag, we use document.getElementById("myButton") to select the button.
- We attach an event listener to the button using .addEventListener(), specifying that the function should be triggered when the click event occurs.
- The function inside the event listener displays an alert with the message Button was clicked!.
Conclusion: #
In this article, you learned two different methods to display a message when a button is clicked in JavaScript.
The first method involves using the onclick attribute within the HTML button element, while the second method utilizes JavaScript event listeners to achieve the same functionality.